#!/usr/bin/python3
# Description: Read and write file using Python.
# open() mode options:
# r = open for reading.
# a = append to the end of the file.
# x = create a file, returns an error if the file exist.
# w = overwrite any existing content.
# Write
# ##########
file = open("my-file.txt", "a")
# Add text to file.
file.write("First line.\n")
file.write("Second line.\n")
file.write("Third line.\n")
# Close file.
file.close()
# Read
# ##########
file = open("my-file.txt", "r")
print(file.read()) # Read the whole file.
# Read each line.
with open("my-file.txt", "r") as file:
for line in file.readlines():
print(line)
# Note: Using 'with' statement will automatically close the file after your are done.
# There is no need to explicitly close the file.
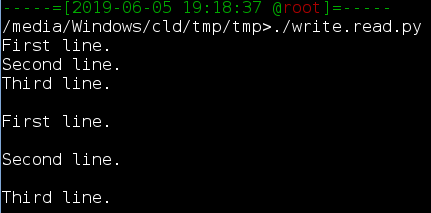
Reference:
- https://docs.python.org/3/library/functions.html#open
- http://docs.python.org/reference/compound_stmts.html#the-with-statement