Install PhpSpreadsheet without composer
- Download PhpSpreadsheet library from https://php-download.com/package/phpoffice/phpspreadsheet.
- Unzip phpoffice_phpspreadsheet_X.X.X.X_require.zip.
- You will get the following files structure:
composer.json
composer.lock
index.php
vendor/
Export Excel file to CSV
<?php
// Load PhpSpreadsheet library.
require_once('vendor/autoload.php');
// Import classes.
use PhpOffice\PhpSpreadsheet\Spreadsheet;
use PhpOffice\PhpSpreadsheet\IOFactory;
// Read the Excel file.
$reader = IOFactory::createReader("Xlsx");
$spreadsheet = $reader->load("my-excel-file.xlsx");
// Export to CSV file.
$writer = IOFactory::createWriter($spreadsheet, "Csv");
$writer->setSheetIndex(0); // Select which sheet to export.
$writer->setDelimiter(';'); // Set delimiter.
$writer->save("my-excel-file.csv");
?>
Output
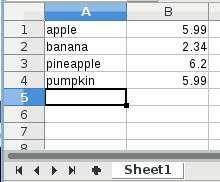
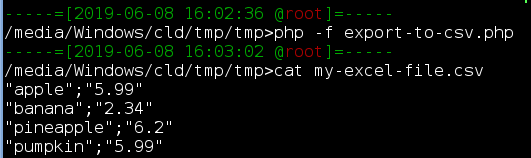
Reference
- List of different type of formats(e.g. XLS, ODS, etc): https://phpspreadsheet.readthedocs.io/en/latest/topics/reading-and-writing-to-file/